Shares and referrals
Share configuration
Shares can be customized by setting the default configuration, or overriding defaults on a per-share basis. Configuration given to an individual share will be merged with the global defaults.
Megacool.Instance.DefaultShareConfig = new MegacoolShareConfig() { Message = "Come play with me!", FallbackImage = "share_fallback.gif",};Megacool.Instance.Share(new MegacoolShareConfig() { // Overrides the default "Come play with me!" set above for // this specific share Message = "Challenge me to a match!", Url = new Uri("/challenge?player=ninja", UriKind.Relative),});
The example above will create a share link that looks like this:
https://mgcl.co/<app-identifier>/challenge?player=ninja&_m=<referral-code>
When a receiver opens the app from the link, a MegacoolShare with the url given
will be included in the MegacoolReceivedShareOpenedEvent
event.
Handling shares and events
Add delegates to LinkClicked
, ReceivedShareOpened
and SentShareOpened
on the
Megacool.Instance
to listen for events emitted from the SDK.
You can implement event listeners to:
- reward your users for referring friends
- send users to a specific location in the app
- automatically make two users friends
Check out the SDK reference docs for the Megacool class and the MegacoolShare to see all fields and methods available.
Receiver opens a shared link
This is an example of how to implement the event listener:
Megacool.Instance.ReceivedShareOpened += (MegacoolReceivedShareOpenedEvent megacoolEvent) => { // This device has received a share to the app and opened it Debug.Log("Got ReceivedShareOpenedEvent: " + megacoolEvent); // Get the user id of the sender string senderUserId = megacoolEvent.SenderUserId; if (megacoolEvent.IsFirstSession) { // First install on this device Debug.Log("First install, invited by user id: " + senderUserId); } else { // The app was already installed on this device Debug.Log("App opened, invited by user id: " + senderUserId); }};// The delegate must be added before calling Start()Megacool.Instance.Start();
Note: This event will not be triggered if you open the link from the same device that you shared the link from.
Sender is notified about an opened link
This is an example of how to implement the event listener:
Megacool.Instance.SentShareOpened += (MegacoolSentShareOpenedEvent megacoolEvent) => { // The receiver of the share has opened it Debug.Log("Got SentShareOpenedEvent: " + megacoolEvent); // Get the user id of the receiver string receiverUserId = megacoolEvent.ReceiverUserId; if (megacoolEvent.IsFirstSession) { // Receiver installed the app for the first time Debug.Log("Receiver installed the app, user id: " + receiverUserId); } else { // The receiver had already installed the app Debug.Log("Receiver opened the app, user id: " + receiverUserId); }};// The delegate must be added before calling Start()Megacool.Instance.Start();
Note: This event will not be triggered if you open the link from the same device that you shared
the link from. Also, the SDK only communicates with the backend occasionally, so this event might
not be triggered immediately. You can force a check for new events by calling
GetShares(Action<List<MegacoolShare>>)
, as that will in addition to getting shares, check for new events.
void RefreshButton() { // This causes a check for new events Megacool.Instance.GetShares(null);}
Link clicked
You can improve the experience for existing users by sending them to the right location in your app immediately after they've opened the link. This is only for users that already have the app installed.
Megacool.Instance.LinkClicked += (MegacoolLinkClickedEvent megacoolEvent) => { // The app has been opened from a link. Send the user instantly // to the right location if the URL path exists. Uri url = megacoolEvent.Url; Debug.Log("Clicked url: " + url); // Assuming you have a way to challenge other players and that // urls are created like "/challenge?player=ninja" you can // implement links that take you straight to the challenge like // this if ("/challenge".Equals(url.AbsolutePath)) { SceneManager.LoadScene("ChallengePlayer"); }};// The delegate must be added before calling Start()Megacool.Instance.Start();
Testing that your referrals work
You can read about how to test your referrals here.
Share state
Call GetShares(Action<List<MegacoolShare>>)
to get the updated state of a sent share. The locally
cached shares will be returned immediately and are useful to determine the total number of shares.
The callback, if given, will receive the up-to-date shares.
A share's ShareState describes how far it has come towards generating an install.
Megacool.Instance.GetShares(shares => { foreach (MegacoolShare share in shares) { if (share.State == MegacoolShare.ShareState.INSTALLED) { Debug.Log("Share sent on " + share.CreatedAt + " created at least one install"); } }});
You can use the share state to display the number of friends that have been invited and what the state is:
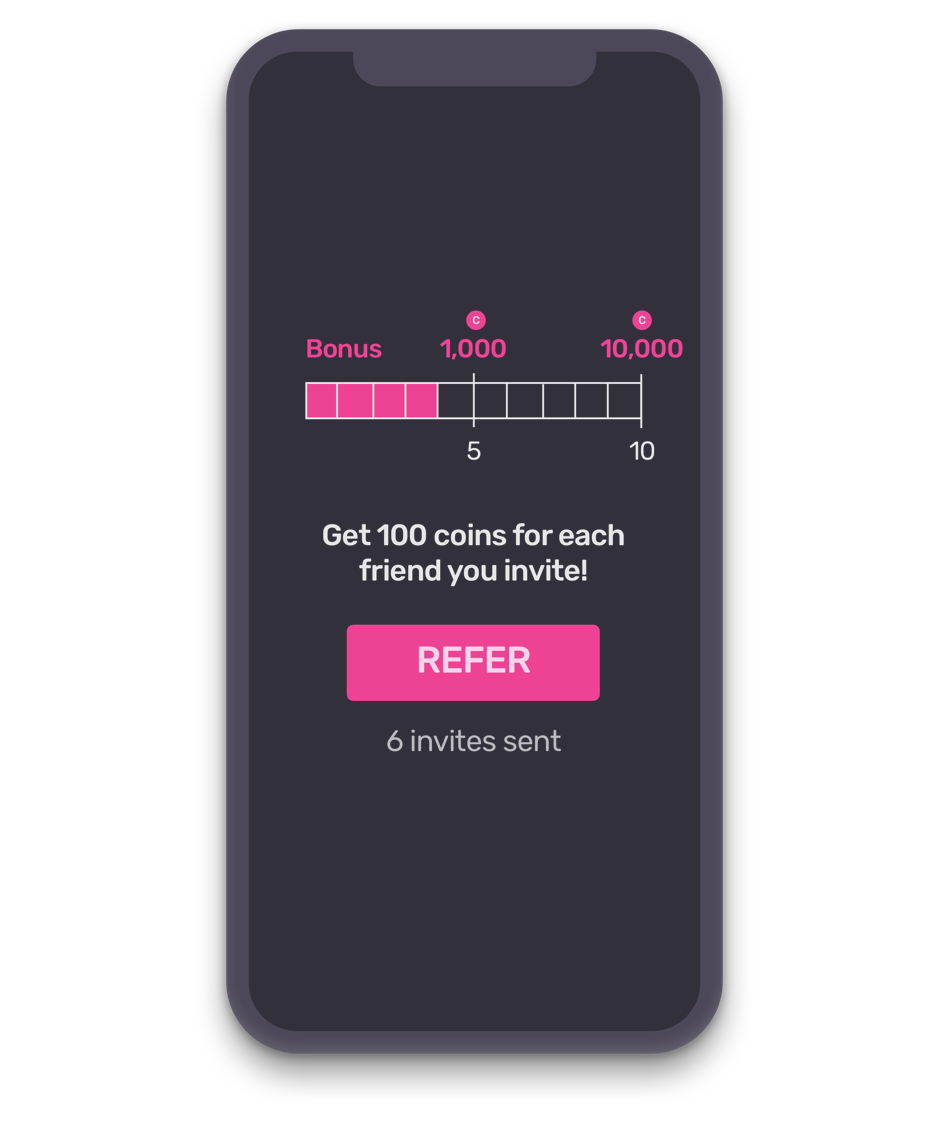
The local share cache doesn't take up much space, but if you want to clean up a bit you can delete shares that match some given criteria. Here's an example that shows how to delete all shares that are older than 1 week:
Megacool.Instance.DeleteShares(share => { return (new DateTime() - share.CreatedAt).TotalDays > 7;});
Share directly to given apps
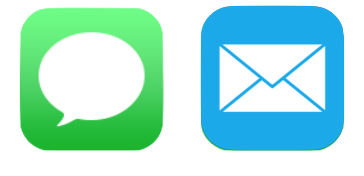
In addition to Megacool.Instance.Share()
, you can also add shortcut buttons for sharing to
specific apps. This reduces the numbers of steps needed to complete a share.
Messages
Megacool.Instance.ShareToMessages();
Megacool.Instance.ShareToMail();
These can also be further customized like described [above](#share-configuration).
Delegates after a share
If you need to dismiss a modal or similar when a share completes, the SDK has some events you can listen to to know when the sharing process ends.
// Don't trust the outcome reported by these callbacks 100%, as some apps// the user can share to don't correctly report the outcome of the shareMegacool.Instance.CompletedSharing += () => { Debug.Log("Share completed successfully");};Megacool.Instance.PossiblyCompletedSharing += () => { Debug.Log("Share might have completed successfully");};Megacool.Instance.DismissedSharing += () => { Debug.Log("Share was dismissed before completion");};
Sharing strategies
Not all apps support sharing both a link and a GIF, and we have to choose which one to include. By default the SDK will prioritize links, to ensure referrals work, but if you only want to ensure the GIFs are spread as widely as possible you can customize this behavior:
Megacool.Instance.DefaultShareConfig = new MegacoolShareConfig() { Strategy = MegacoolSharingStrategy.MEDIA,};